728x90
반응형
#cv2.findContours(image, mode, method): 이미지에서 Contour들을 찾는 함수
#mode: Contour들을 찾는 방법
#1) RETR_EXTERNAL: 바깥쪽 Line만 찾기
#2) RETR_LIST: 모든 Line을 찾지만, Hierarchy 구성 X
#3) RETR_TREE: 모든 Line을 찾으며, 모든 Hierarchy 구성 O
#method: Contour들을 찾는 근사치 방법
#1) CHAIN_APPROX_NONE: 모든 Contour 포인트 저장
#2) CHAIN_APPROX_SIMPLE: Contour Line을 그릴 수 있는 포인트만 저장
#입력 이미지는 Gray Scale Threshold 전처리 과정이 필요합니다.
#cv2.drawContours(image, contours, contour_index, color, thickness): Contour들을 그리는 함수
#contour_index: 그리고자 하는 Contours Line (전체: -1)
import cv2
import matplotlib.pyplot as plt
image = cv2.imread('cat.jpg')
image_gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(image_gray, 127, 255, 0)
plt.imshow(cv2.cvtColor(thresh, cv2.COLOR_GRAY2RGB))
plt.show()
contours = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)[0]
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 4)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()

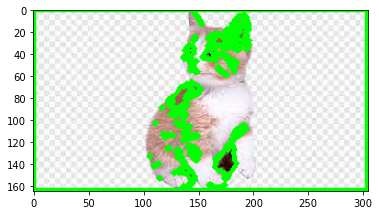
import cv2
import matplotlib.pyplot as plt
image = cv2.imread('cat.jpg')
image_gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(image_gray, 127, 255, 0)
plt.imshow(cv2.cvtColor(thresh, cv2.COLOR_GRAY2RGB))
plt.show()
contours = cv2.findContours(thresh, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)[0]
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 4)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()


import cv2
import matplotlib.pyplot as plt
image = cv2.imread('cat.jpg')
image_gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(image_gray, 127, 255, 0)
plt.imshow(cv2.cvtColor(thresh, cv2.COLOR_GRAY2RGB))
plt.show()
contours = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[0]
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 4)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()


import cv2
import matplotlib.pyplot as plt
image = cv2.imread('cat.jpg')
image_gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
#threshold로 이진법화 하였고, 127보다 높은 값은 255로 설정하고, 아니면 0으로 설정
ret, thresh = cv2.threshold(image_gray, 150, 255, 0)
plt.imshow(cv2.cvtColor(thresh, cv2.COLOR_GRAY2RGB))
plt.show()
contours = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_NONE)[0]
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 4)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()
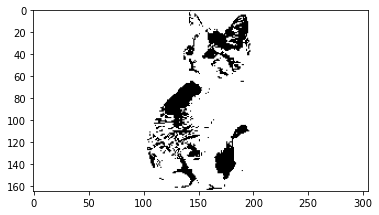

728x90
반응형
'Data Analysis > Data Analysis & Image Processing' 카테고리의 다른 글
12. OpenCV Filtering (0) | 2022.04.16 |
---|---|
11. OpenCV Contours 처리 (0) | 2022.04.16 |
9. OpenCV 도형 그리기 (0) | 2022.04.16 |
8. OpenCV Tracker (0) | 2022.04.16 |
7. OpenCV 임계점 처리하기 (0) | 2022.04.07 |
댓글